Science Computer (SC025)
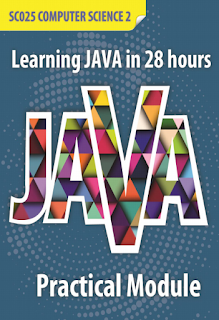
-This module will give you a very brief explaination about How To Learn Java π https://drive.google.com/open?id=1gYJSdni2mYNRFlkqVXBTKHMz5fygK-dd
(A) Notes
(B) Exercise
(C) Simple Revision Notes
TOPIC 1 : APPROACH IN PROBLEM SOLVING
Chapter 2 β€DESIGN SOLUTION
(A) Notes
(B) Exercise
https://drive.google.com/open?id=1HTa8l9m7rIfxdN_pbYaPpXh9zCGEi0WP
Technique To Answer Repetition Question
TOPIC
2 : DESIGN A SOLUTION
v ALGORITHMS :-
Well defined procedure that allows a computer to solve a problem
Ways to present :-
(A) Pseudocode
(B) Flowchart (Graphical representation of problem solving)
v Pseudocode :-
(A) Semiformal, English-like language with a limited vocabulary that can be
used to design an algorithm
(B) Purpose : To define the procedural logic of an algorithm in a simple and
easy to understand for readers who are not proficient in computer programming
language.
v Control Structure :-
(A) Block of programming that analyse variable and choose direction based on
given parameters.
(B) Sequence, Selection and Repetition
(C) Sequence : All actions will be executed sequentially
(D) Selection :-
(i) Single Selection
(ii) Dual Selection
(iii) Multiple Selection
*there can be if without else but there
cannot be else without if
(E) Repetition :-
(i) will be represented by using
keyword while
(ii) Counter-controlled repetition
(1) Initialization : Set the
initial value of the loop control variable
(2) Evaluation : Test the
loop control variable to determine whether the loop
should
continue or stop
(3) Updating : Increment /
Decrement the value of the loop control variable
(iii) Loop Control Variable
: Variable that control the
number of times the loop is executed
(iv) Sentinel-controlled repetition
: number of loop is NOT known before loop
begins executing
: the loop will stop when
sentinel value is reached
(F) Desk Checking :
(i) Informal manual test that
programmers can use to verify coding
(ii) Enable to spot errors that
might prevent a program from working as it should
(A) Notes
https://drive.google.com/open?id=1FkJdn-yOZRMd0AMzfcdPPyMUwQMnn87_
(B) Exercise
https://drive.google.com/open?id=1AysGrsioz0TLCMUEwxfe805h0eEfT9I6
(C) Simple Revision Notes
3.1 INTRODUCTION TO JAVA
Object-Oriented Program (OOP)
- A programming technique that uses objects to design the applications and computer programs.
(1) Characterictics:-
(a) Abstraction
- Is a process of hiding the implementation details and showing only functionality to the user
- Purpose : Allow efficient design and implementation of complex software systems.
(b) Encapsulation
- Is a process of wrapping code and data together into a single unit
- Purpose : Wrap its inner attributes from outside the object
: Allow behaviors to be accessible outside the object
(c) Inheritance
- Is a mechanism in which one object acquires all the properties and behaviors of a parent object
- Purpose : Itβs the ability to create a new class from an existing class
(d) Polymorphism
- Is the ability to appear in many forms
- Purpose : Helps programmers reuse the code and classes once written, tested and implemented
(2) Class
- Template or blueprint to create an object
(3) Object
- Is an identifiable entity with some characteristics and behavior
- It is an instance of a class
- Objects are specific and created from classes
3.1 DATA TYPE, OPERATOR AND EXPRESSION
(A) Identifier
- Words used to name constants, variables and functions
- Identifiers are formed by combining letters, digits and underscore
- Words used to name constants, variables and functions
- Identifiers are formed by combining letters, digits and underscore
VALID
|
INVALID
|
income_tax
|
Student age
|
Item10
|
17throw
|
Count
|
Data-1
|
Number_of_students
|
Myfirst.c
|
ABC123Z7
|
12
|
(B) Variable
- Name given to the memory location where a value stored
(C) Constant
- Is an identifier that similar to a variable except that it holds one value while the program is active
(D) Primitive Data Types
Keyword
|
Description
|
Example
|
Java Statement
|
int
|
Integer
|
2, 4, 100
|
sc.nextInt( );
|
double
|
Floating point
|
74.5, 12.45
|
sc.nextDouble( );
|
boolean
|
Boolean value
|
True or False
|
sc.next( );
|
char
|
Single Character
|
1, A, 2
|
sc.next( ).charAt(0);
|
String
|
name
|
Nur izwani
|
sc.nextLine( );
|
(E) Operators
- Is a symbol which tells the compiler to take an action on operands and yield a value
Arithmetic Operation
|
Aritmetic Operator
|
Java Statement
|
Addition
|
+
|
a+b;
|
Subtraction
|
-
|
a-b;
|
Multiplication
|
*
|
a*b;
|
Division
|
/
|
a/b;
|
Modulus(Remainder)
|
%
|
a%b;
|
(F) Relational Operators
- To test the relation between two values
- To test the relation between two values
Relational Operation
|
Relational Operator
|
Equal to
|
= =
|
Less than
|
<
|
More than
|
>
|
Less than or equal
|
<=
|
More than or equal
|
>=
|
Not equal
|
!=
|
(G) Precedence
Operator
|
Precedence
|
|
( )
|
πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ πΊ
πΊ
Lowest |
|
!
|
||
*
|
||
/
|
||
% (Remainder)
|
||
+
|
||
-
|
||
<
|
||
<=
|
||
>
|
||
>=
|
||
= =
|
||
!=
|
||
&& (and)
|
||
| | (or)
|
||
=
|
(H) Escape Characters
- Sequence begins with a backslash / followed by a character
Escape Sequence
|
Meaning
|
\n
|
A new line
|
\t
|
A tab
|
\β
|
A double square
|
\β
|
A single quote
|
\\
|
Backslash
|
\b
|
Backspace
|
3.2 ARRAY
Array
- A data structure that contains a group of elements.
- Purpose : To store multiple value of the same type using single variable
: Enable you to write programs that manipulate larger amounts of data
(i) Declare A Variable
WITH BASIC TYPE
|
WITH AN ARRAY TYPE
|
int counts;
|
int [ ] counts;
|
(ii) Line of Code
DOUBLE LINE OF CODE
|
SINGLE LINE OF CODE
|
double [ ] values;
values = new double [7]; String [ ] name; name = new String[7]; int [ ] num; num = new int[7]; |
double [ ] values = new
double [7];
-------------------------------------------------
String [ ] name = new string [7];
-------------------------------------------------
int [ ] num = new int [7];
-------------------------------------------------
|
(iii) Reserved Word
: new/ return/ void/ int/ double/ boolean
3.3 METHODS
Method
- A component of a program/small program written to perform a specific task
- Advantage :-
(i) Code reusability
: Code written once can be used many times anywhere in the program
(ii) Make code more readable and easier to debug
: Segregrated into different smaller modules, locating the errors and correct easily
Type of method
(a) Standard Library Methods
- built-in methods in java and part of the compiler package
Method
- A component of a program/small program written to perform a specific task
- Advantage :-
(i) Code reusability
: Code written once can be used many times anywhere in the program
(ii) Make code more readable and easier to debug
: Segregrated into different smaller modules, locating the errors and correct easily
Type of method
(a) Standard Library Methods
- built-in methods in java and part of the compiler package
Math.sqrt(x);
|
String charAt(index),
length ( )
|
Math.pow(x,y);
|
System methods- print( ),
println( )
|
Scanner methods- next( ),
nextInt( ), nextDouble( ), nextBoolean.
|
(b) User β Defined Methods
- Created by the programmer.These methods take-on names that you assign to
them and perform tasks that you create
Modifier
|
Define
access type of the method and optional to use ( public static / private
static )
|
Return
Type Value
|
Method
may return a value
|
Method
Name
|
Method
signature consists of the method name and the parameter list
|
Parameters
|
Variables
in the method definition
|
Method
Body
|
Defines
what the method does with the statements
|
(c) Class Name : The program class name
(d) Object Name : The instance of the class
(object for the class name)
Method Return Type
(a) Do not return a value
: uses the keyword void as return type in its heading to show that it does not
return a value
(b) Return a value
: returns a value must specify the type of that value in its heading
Argument
|
Parameters
|
- A value
we pass to a method
|
- The
variables in the method definition
|
Practical Notes (Array And Method)
β€β€https://drive.google.com/open?id=1RSq8VegYcbh_KeCXCYBaHV9Qpg2GtmIL
Youtube Video (Array)
β€β€https://youtu.be/w0_W5oDKQYI
Trial Set Paper 19/20
β€β€https://drive.google.com/open?id=1R1JBk0JzSBG5fmSCgGbghaH3ptHkyphA96 Java Coding
(Selection, Sequence, Repetition, Array, Method)
β€β€https://drive.google.com/open?id=1-a8tUifwnrFow09K6_F-gxrzD97fFHKh
COMPLETED !ππͺ
Comments
Post a Comment